Design patterns are all about reusable solutions to common problems in software designing that occur in real-world application development.
The Factory Method design pattern is one of the twenty-three well-known “Gang of Four” design patterns that describe how to solve recurring design problems to design flexible and reusable object-oriented software, that is, objects that are easier to implement, change, test, and reuse.
The Factory Method design pattern solves problems like:
- How can an object be created so that subclasses can redefine which class to instantiate?
- How can a class defer instantiation to subclasses?
Creating an object directly within the class that requires (uses) the object is inflexible because it commits the class to a particular object and makes it impossible to change the instantiation independently from (without having to change) the class.
The Factory Method design pattern describes how to solve such problems:
- Define a separate operation (factory method) for creating an object.
- Create an object by calling a factory method.
Here we took and example as Remote Student Support Tool. It’s have below 4 methods like
- ReceiveRequest
- GenerateSupportSession
- StartMeeting
- EndMeeting
For remote support we can use three remote supporting tool.
- Goto Meeting
- WebEx Meeting
- Team Viewer
To create project follow the below steps

Step 1: click on New project in visual studio.
Step2:
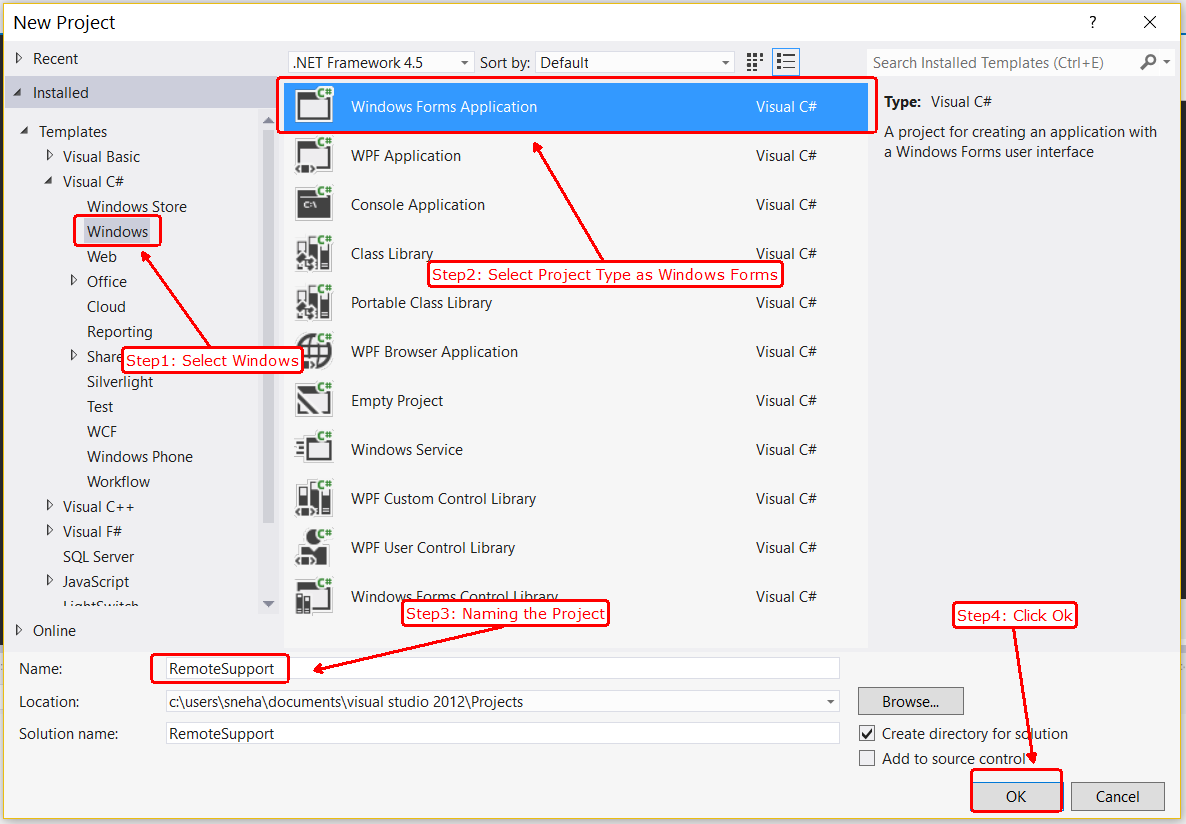
Before start to code, we need to select the platform, what is the programming language we have to implement. For this demo I have used C# language and Windows application.
You can use any language like Vb, C++ or F#.
After creating the project, the first step we need create class Library, for code reusability and readability I prefer to all interface and business logic into separate class library.
Here, I have created SupportBusinessAcessLayer and place all interface, classes and Factory Classes in this project. Simply I just refer this class library to my window application.
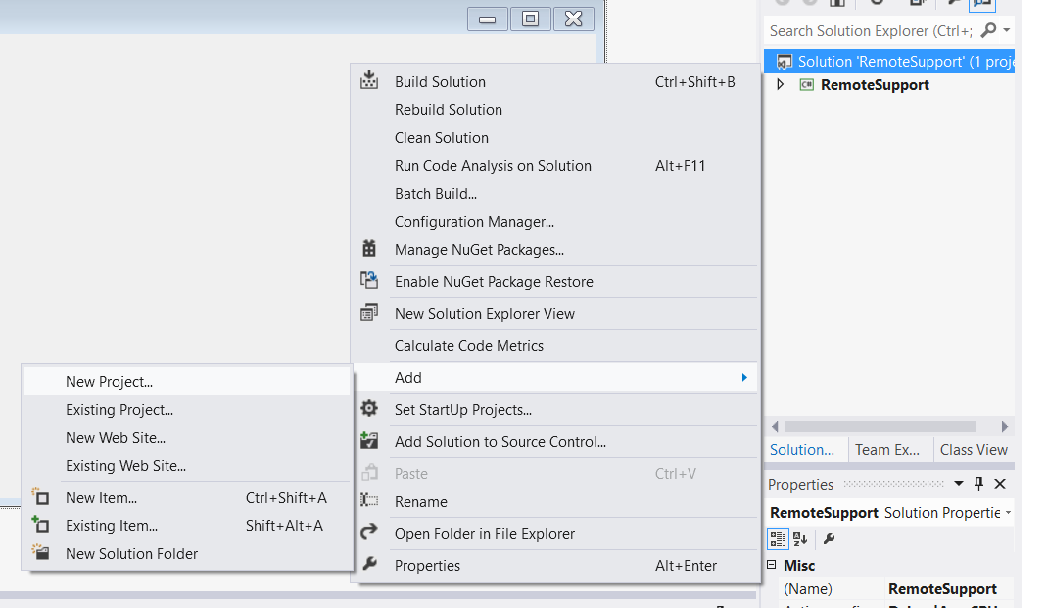


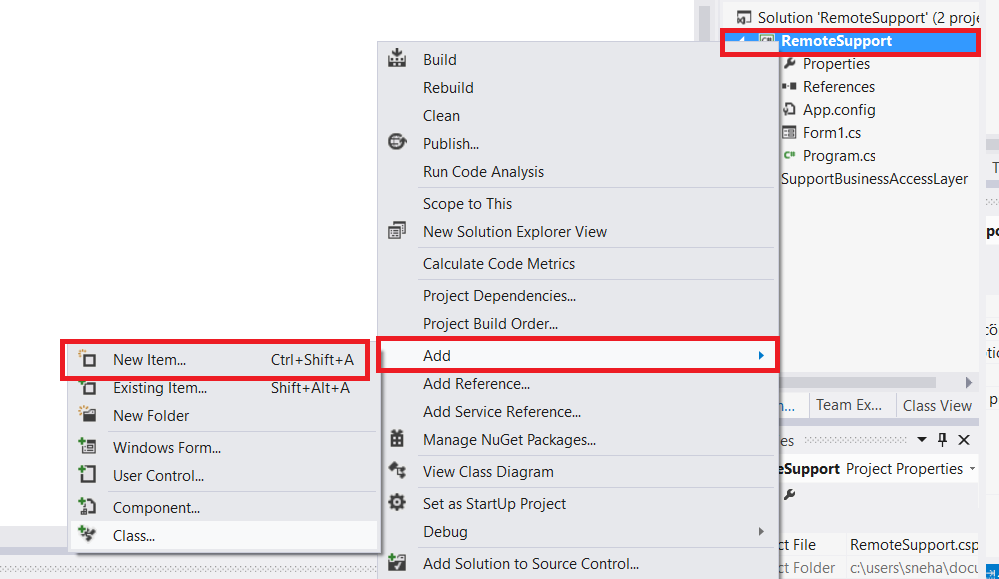

After create the IConnect interface, we have to define the basic method which can be used across all tools.
Here I have used Interface for this, if you have a situation, like few methods is not mandatory to implement then we go for abstract class.
public interface IConnect
{
string ReceiveRequest(int StudentId);
string GenerateSupportSession(out string MeetingId);
string StartMetting(string MeetingId);
string EndMeeting(string MeetingId);
}
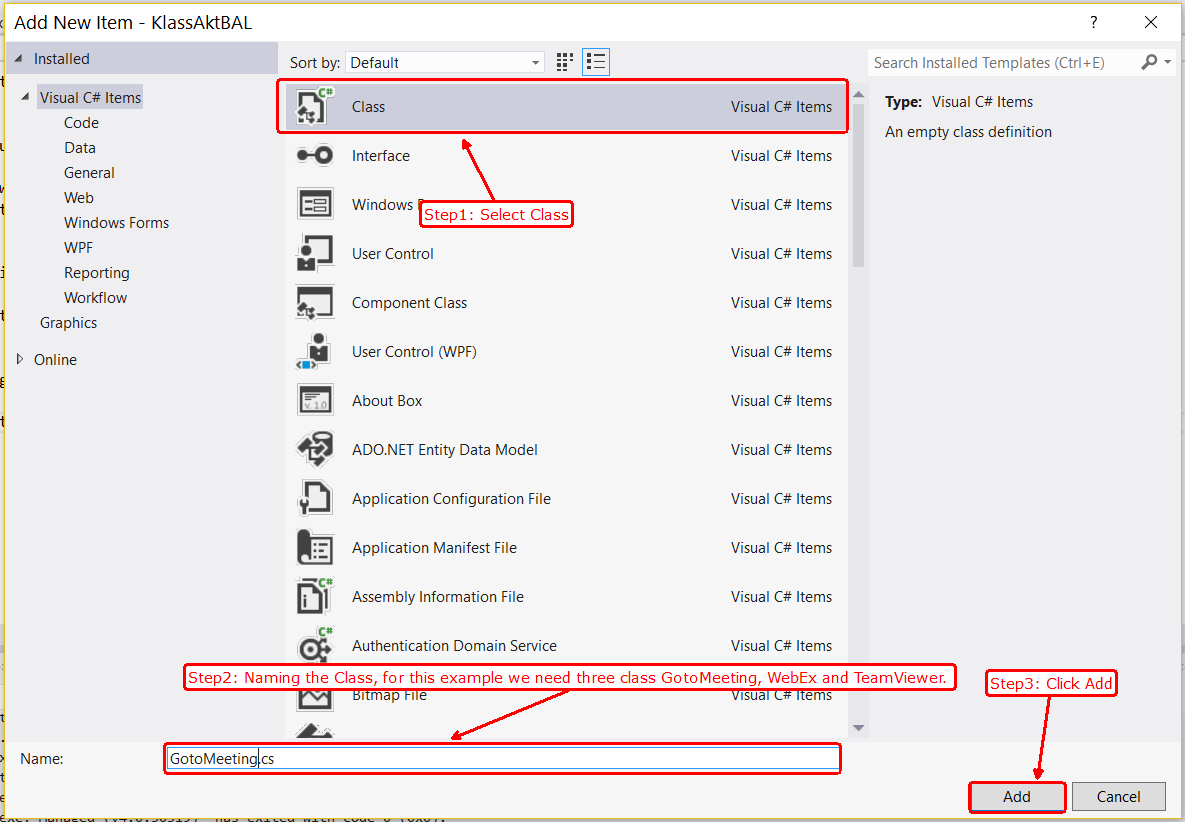

Goto Meeting Class
class GotoMeeting : IConnect
{
public string ReceiveRequest(int StudentId)
{
return string.Format("Goto: Receive request from Student Id: {0}", StudentId);
//here we need to write logic to receive request from student.
}
public string GenerateSupportSession(out string MeetingId)
{
MeetingId = Guid.NewGuid().ToString();
return string.Format("Goto: Created Session. Session Id {0}", MeetingId);
//here we need to generate goto session using Goto meeting API. for demo purpose i have created Guid and return back via out parameter.
}
public string StartMetting(string MeetingId)
{
return string.Format("Goto: Started Meeting using {0} meeting ID.", MeetingId);
//here we need to implement the logic to start the meeting in goto meeting API
}
public string EndMeeting(string MeetingId)
{
return string.Format("Goto: Meeting ended of {0} Id.", MeetingId);
//end the meeting using meeting id
}
}
WebEx Class
class WebEx : IConnect
{
public string ReceiveRequest(int StudentId)
{
return string.Format("WebEx: Receive request from Student Id: {0}", StudentId);
}
public string GenerateSupportSession(out string MeetingId)
{
MeetingId = Guid.NewGuid().ToString();
return string.Format("WebEx: Created Session. Session Id {0}", MeetingId);
}
public string StartMetting(string MeetingId)
{
return string.Format("WebEx: Started Meeting using {0} meeting ID.", MeetingId);
}
public string EndMeeting(string MeetingId)
{
return string.Format("WebEx: Meeting ended of {0} Id.", MeetingId);
}
}
Team Viewer
class TeamViewer : IConnect
{
public string ReceiveRequest(int StudentId)
{
return string.Format("TeamViewer: Receive request from Student Id: {0}", StudentId);
}
public string GenerateSupportSession(out string MeetingId)
{
MeetingId = Guid.NewGuid().ToString();
return string.Format("TeamViewer: Created Session. Session Id {0}", MeetingId);
}
public string StartMetting(string MeetingId)
{
return string.Format("TeamViewer: Started Meeting using {0} meeting ID.", MeetingId);
}
public string EndMeeting(string MeetingId)
{
return string.Format("TeamViewer: Meeting ended of {0} Id.", MeetingId);
}
}
Connect Factory
public class ConnectFactory
{
static public IConnect CreateObject(string ConnectType)
{
IConnect obj = null;
switch (ConnectType)
{
case "Goto":
obj = new GotoMeeting();
break;
case "WebEx":
obj = new WebEx();
break;
case "TeamViewer":
obj = new TeamViewer();
break;
default:
break;
}
return obj;
}
}

public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnConnect_Click(object sender, EventArgs e)
{
if (txtOutput.Text != "")
{
txtOutput.Text += "----------------------------------------------------------------------";
txtOutput.Text += Environment.NewLine;
}
IConnect obj = null;
string MeetingId = string.Empty;
obj = ConnectFactory.CreateObject(cmbConnectTool.Text);
txtOutput.Text += obj.ReceiveRequest(int.Parse(txtStudentId.Text));
txtOutput.Text += Environment.NewLine;
txtOutput.Text += obj.GenerateSupportSession(out MeetingId);
txtOutput.Text += Environment.NewLine;
txtOutput.Text += obj.StartMetting(MeetingId);
txtOutput.Text += Environment.NewLine;
txtOutput.Text += obj.EndMeeting(MeetingId);
txtOutput.Text += Environment.NewLine;
}
}
You can see here, I have create the type of object obj as IConnect , I have not mentioned the object class. Based on the user input drop down box, it will call ConnectFactory.CreateObject method with the parameter as Drop down value.
The below are the output of this program.
Final Output: Sample Demo Download here
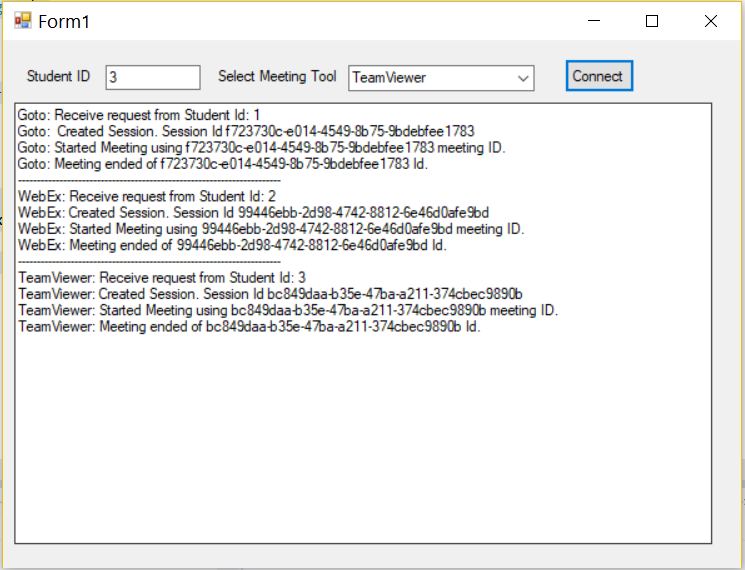